1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58
//! Clustering algorithms. //! //! 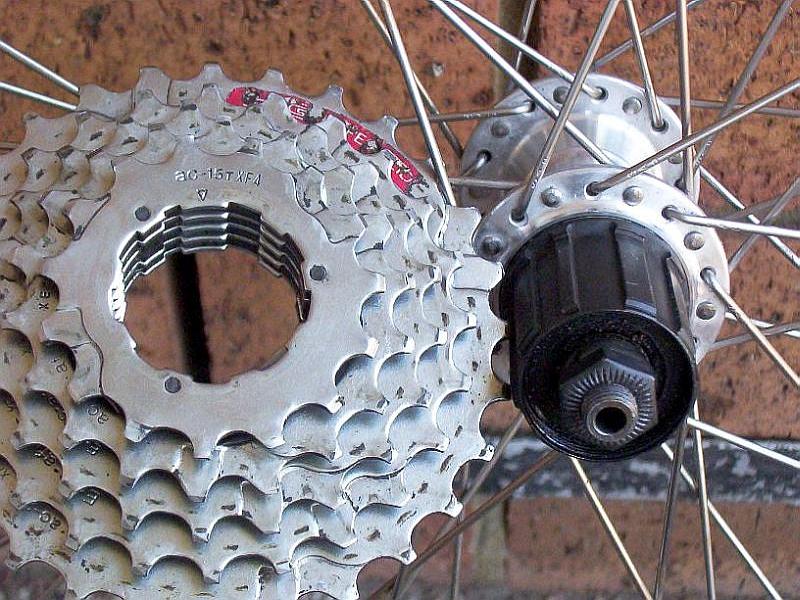 //! //! This crate provides generic implementations of clustering //! algorithms, allowing them to work with any back-end "point //! database" that implements the required operations, e.g. one might //! be happy with using the naive collection `BruteScan` from this //! crate, or go all out and implement a specialised R*-tree for //! optimised performance. //! //! Density-based clustering algorithms: //! //! - DBSCAN (`Dbscan`) //! - OPTICS (`Optics`) //! //! Others: //! //! - *k*-means (`Kmeans`) //! //! [Source](https://github.com/huonw/cogset). //! //! # Installation //! //! Add the following to your `Cargo.toml` file: //! //! ```toml //! [dependencies] //! cogset = "0.2" //! ``` #![cfg_attr(all(test, feature = "unstable"), feature(test))] #[cfg(all(test, feature = "unstable"))] extern crate test; #[cfg(test)] extern crate rand; extern crate order_stat; #[cfg(all(test, feature = "unstable"))] #[macro_use] mod benches; #[cfg(not(all(test, feature = "unstable")))] macro_rules! make_benches { ($($_x: tt)*) => {} } mod dbscan; pub use dbscan::Dbscan; mod optics; pub use optics::{Optics, OpticsDbscanClustering}; mod point; pub use point::{Point, RegionQuery, Points, ListPoints, BruteScan, BruteScanNeighbours, Euclid, Euclidean}; mod kmeans; pub use kmeans::{Kmeans, KmeansBuilder};